Gamma 19: April 2022
Reworking the Player!
In the upcoming chapters of Gamma 19 we want to introduce new systems that the player will use throughout the game. For example, in chapter 1 the player will be given the ability to slide and dodge attacks. While trying to brainstorm some ideas as to how I was going to implement this system in the existing player code I noticed a glaring issue. The code had become unmanageable and impossible to add any new mechanic to it without breaking something. This isn't the first time something like this has happened. During the development of Suits: Absolute Power (Available on Steam for $4.99) this was a common issue when developing many systems as I was still learning the engine. The player code was the first thing in the Godot version and it shows. Here is a snippet of code that handles the animation logic (Click on the image to expand it):
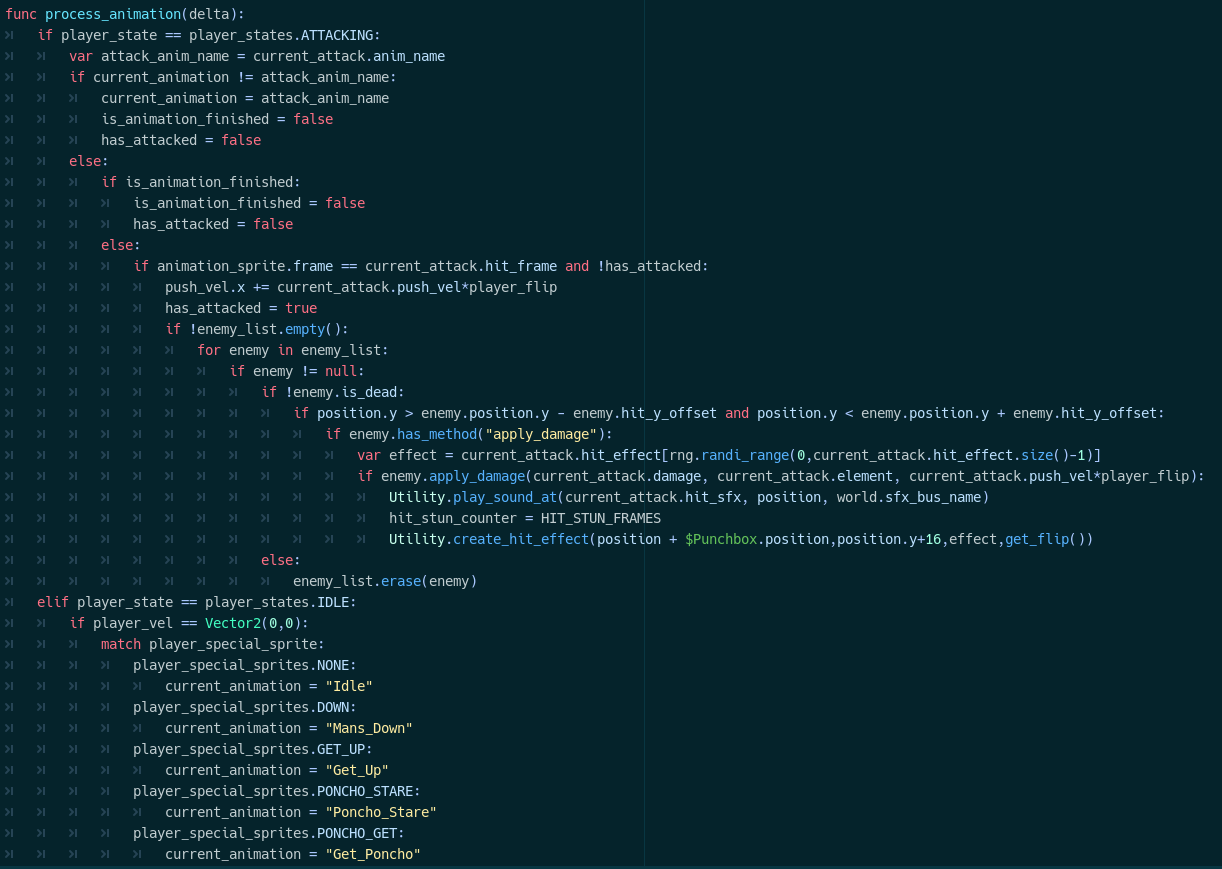
There were a few problems with this code. This code handles both the logic as an animation plays and when it finishes. There is another function
that is called _on_AnimatedSprite_animation_finished()
which was generated by Godot when I connected the signal from the
animated sprite object that triggers every time the animation finishes. In the _on_AnimatedSprite_animation_finished()
function the variable is_animation_finished
is set to true and processes some other checks too. This is a really bad implementation because
you want to keep all of the code that should run when the animation is finished in one function, not in two. The process_animation()
function should only handle any code that should execute while the animation is playing. The other glaring issue is the poor implementation of
a state machine. Basically the idea is that the player will have different states that will change depending on input and other conditions. This
is a very basic implementation of that idea by using if and switch statements to check the state, however, the more complex the code gets the harder
it is to add and change the code as now you have to add another if statement to check for a new state and make sure to add it wherever else it is
needed. Also with this implementation you can change to any state without knowing what the last state was and if you can switch to the next state.
Let's say you wanted to make the player run. The logic would look like this: Idle -> Run
. Simply add another if statement to all functions that check if
we are running. Now we want the player to slide only when we are running: Idle -> Run -> Slide
. Again add another if statement to all functions to check
for this state. Repeat this process for every state and function and now your code is a mess. So how do we fix this?
Finite State Machines
State machines son.
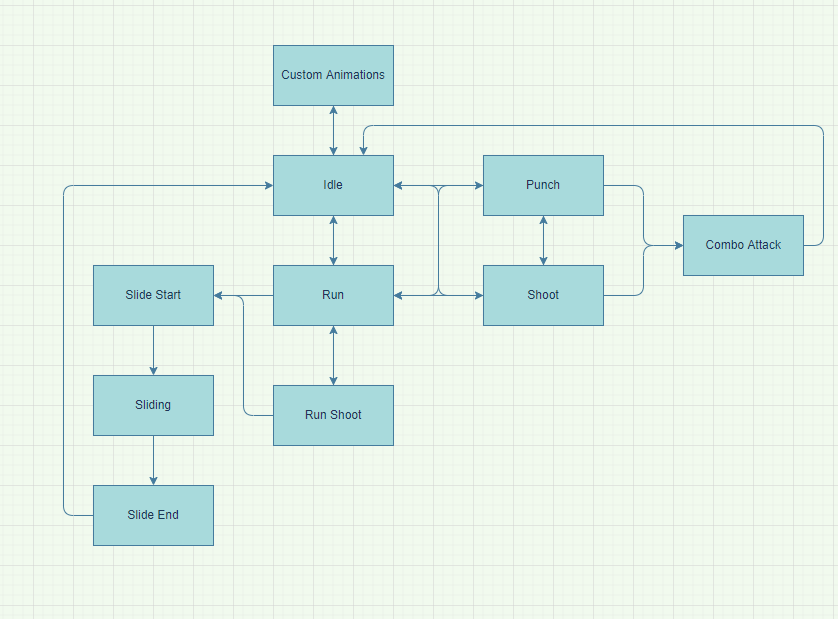
A finite state machine is a design pattern in which each state is broken up into separate objects that will hold their own variables and functions. A state machine object will hold all of the states and only execute the code of the current selected state. That way code from other states won't affect the current state. If you look at the diagram we start at the idle state. From there we can only change to four other states: Run, Punch, Shoot, and Custom Animation. We also have a death state, but it wasn't added in this diagram. Notice how the player can only slide if they are running. Let's take a look at this in Godot.
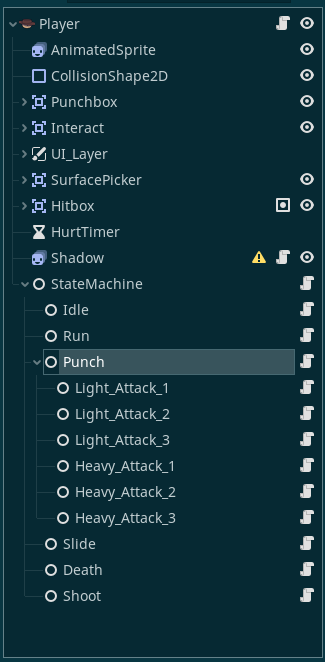
This is the Object tree which holds all of the parts that make up the player object. The StateMachine
node is the object that holds every state for the player. By default the
state machine starts at the Idle state. We call the function transition_to(state, msg)
whenever we need to switch states. It takes two
arguments: state
which is just the name of a state node (Ex: "Idle") and msg
which is a data structure that can hold data for the state to process if needed. Let's take a look
at the StateMachine
code.
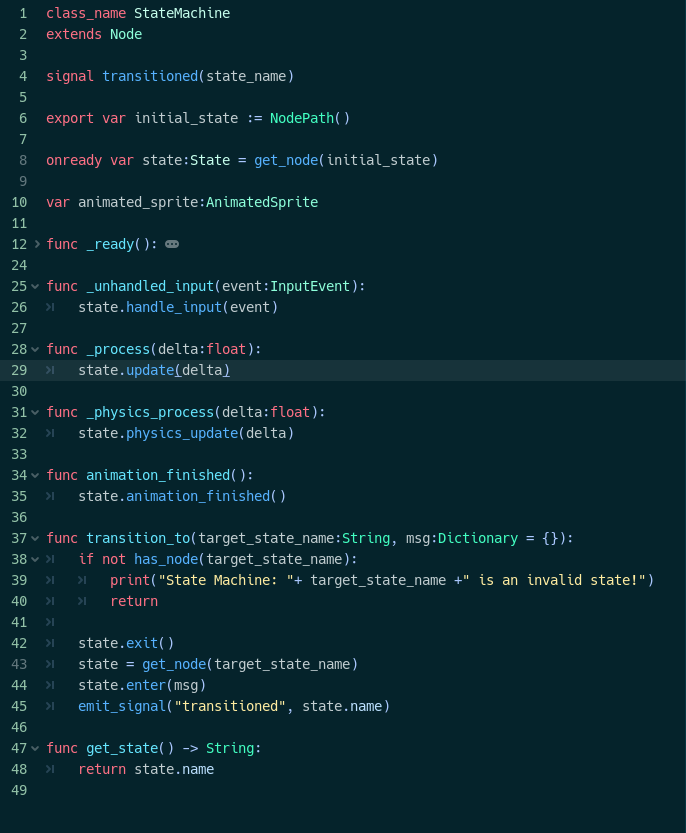
It's relatively simple for such a powerful tool (Even if you don't know GDScript). I'll break down the important details for you. Starting from the top, we
have a variable called state
which holds the current state the player is in. The value of the default state is loaded in when
the code is initialized. The _ready()
function just loops through all of the states and initializes them. Next we have four functions (3 of which are built-in),
_unhandled_input(event:InputEvent)
, _process(delta)
, _physics_process(delta)
, and animation_finished()
. You'll
notice that each of these functions have one line state.some_function()
. This calls a function in the current state. The built-in function _process(delta)
and _physics_process(delta)
tell the engine to automatically run them without needing to be called. So in our State
code we avoid using these built-in
functions so that we don't execute the wrong code.
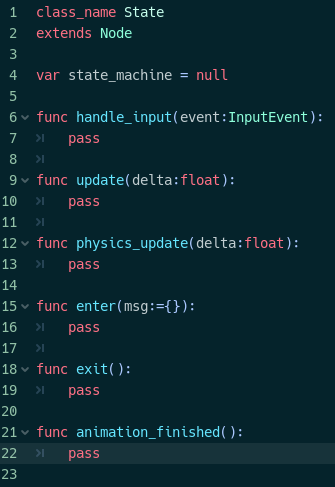
This is a very simple and generic class that we can use in other parts of our game.
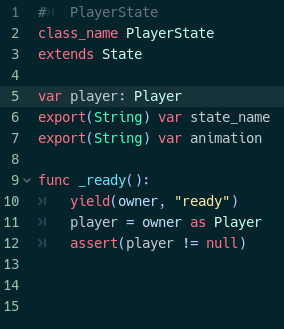
For the player we will be using a custom state that extends from the base state class. Later we will be doing this when we rework the enemies.
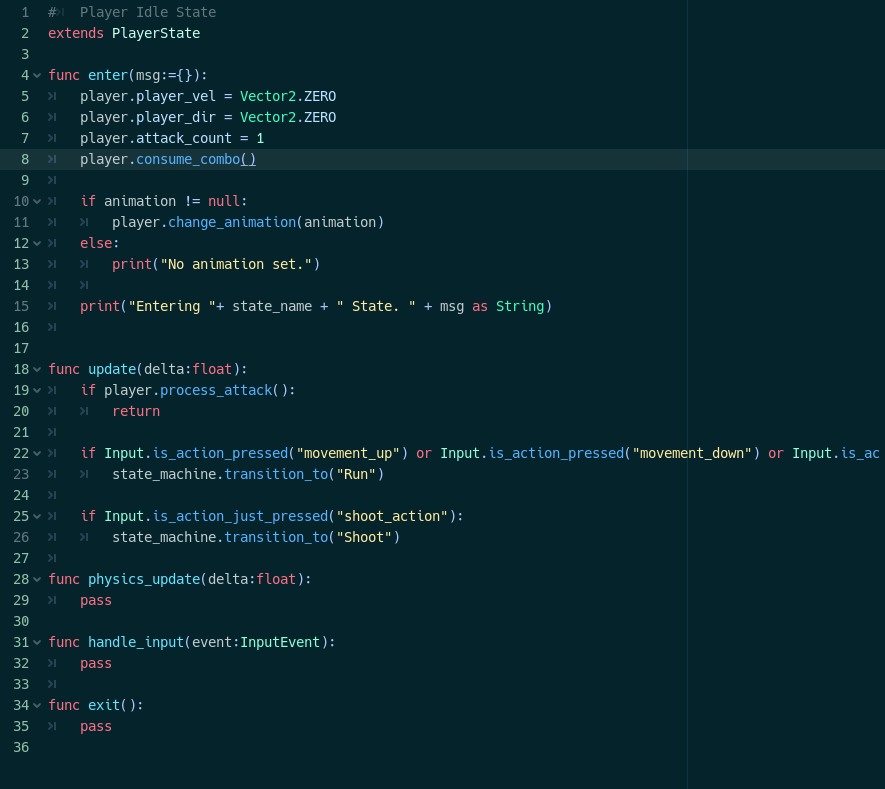
Here is the player idle state code. We override the functions from the base class to do only what we need them to do. The enter(msg:={})
function is executed when we switch to this state and it will reset some values from the player and change the current animation to the player idle
animation. In the update(delta:float)
function we only want to check if the player is going to punch, run, or shoot. If we tried pressing
the slide button nothing would happen because we would need to be in the run state to handle that input.
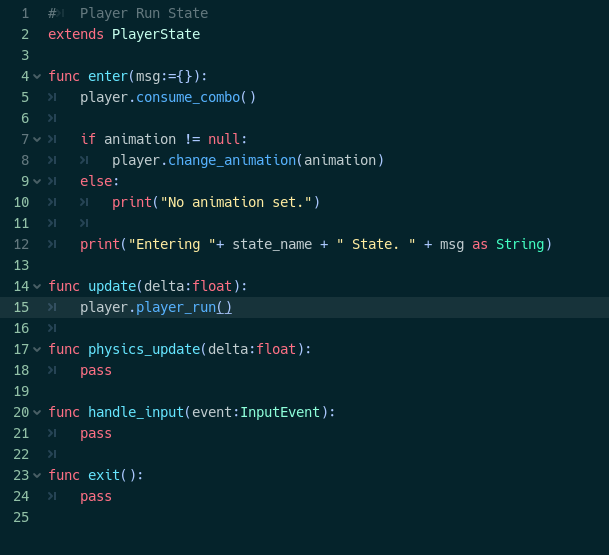
Similar to the idle state, our run state executes the enter(msg:={})
function when we switch to it and the update(delta:float)
function is then executed every frame. In the update(delta:float)
function we call the player function player_run()
which will
handle our input for running. The running input used to be handled only in the run state, but with the introduction of the shoot and run mechanic we moved
that code into the player to reduce redundant code.
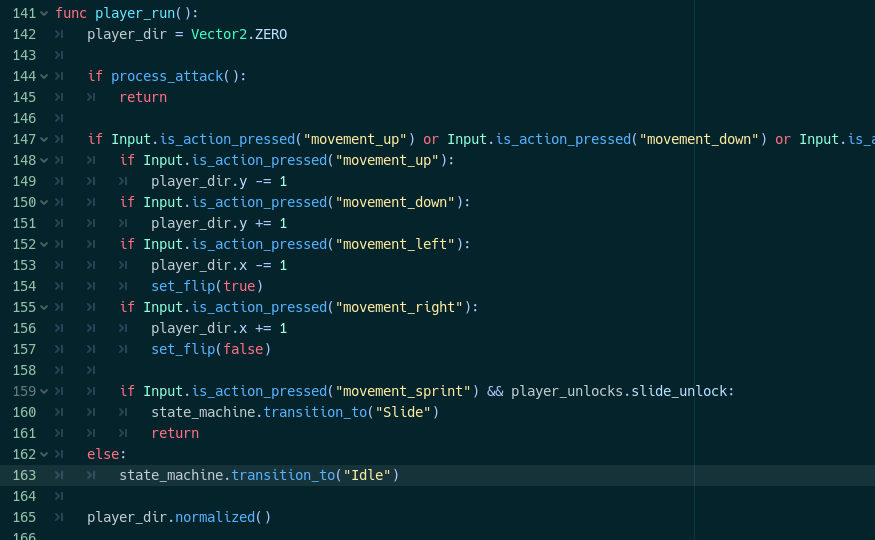
Jumping back to the player code, we can see what the function player_run()
is actually doing. It first checks to see if the player is going to
attack with the process_attack()
function and if they are then it jumps out of the player_run()
function and switches to the punch
state. Otherwise it checks the player input to see if they are moving around, if not it switches back to the idle state.
Art Corner
Not just Suits Art.
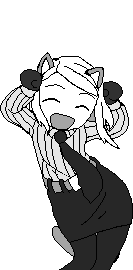
Suits Cat Girl by【book】.
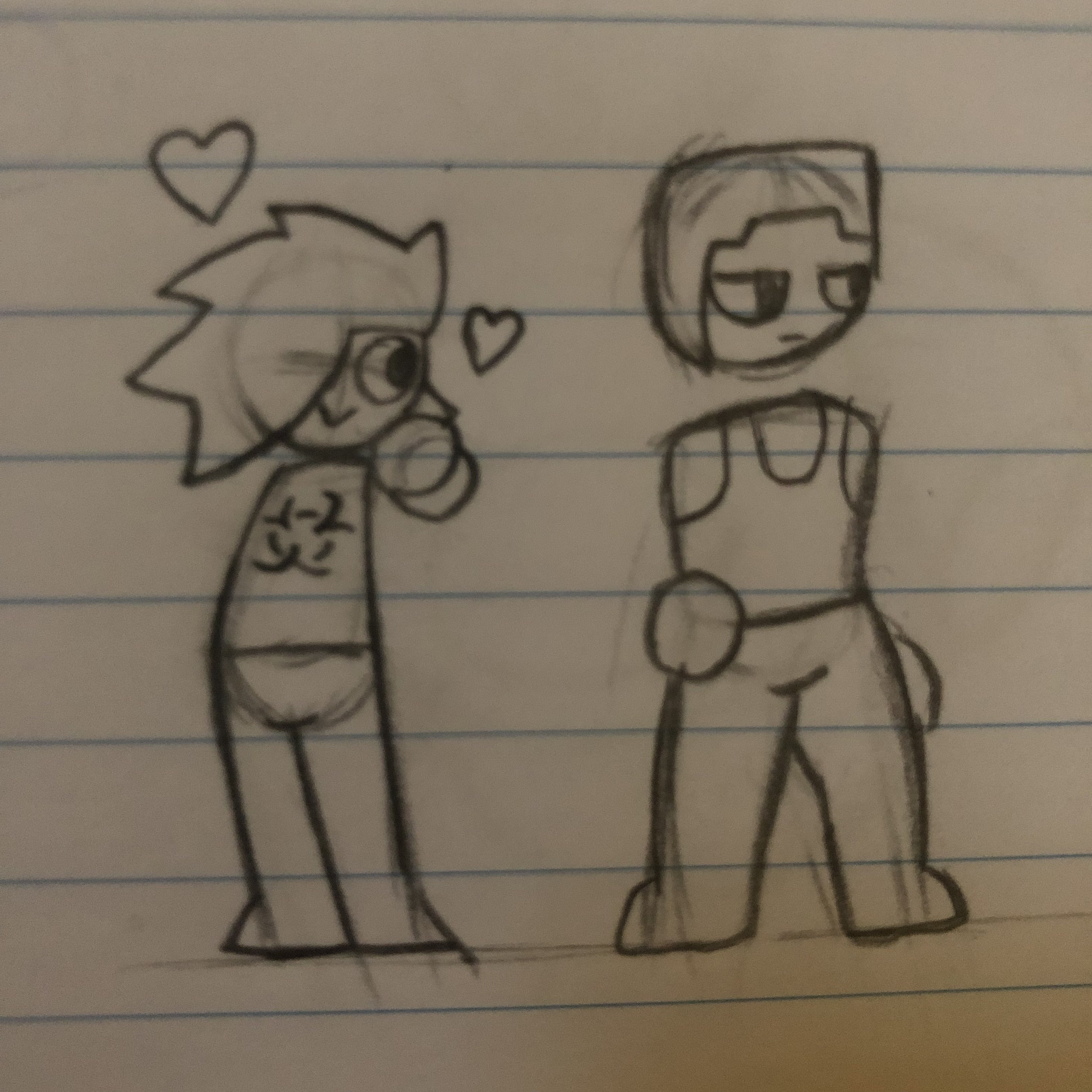
Gay people by W3Rn1ckz.
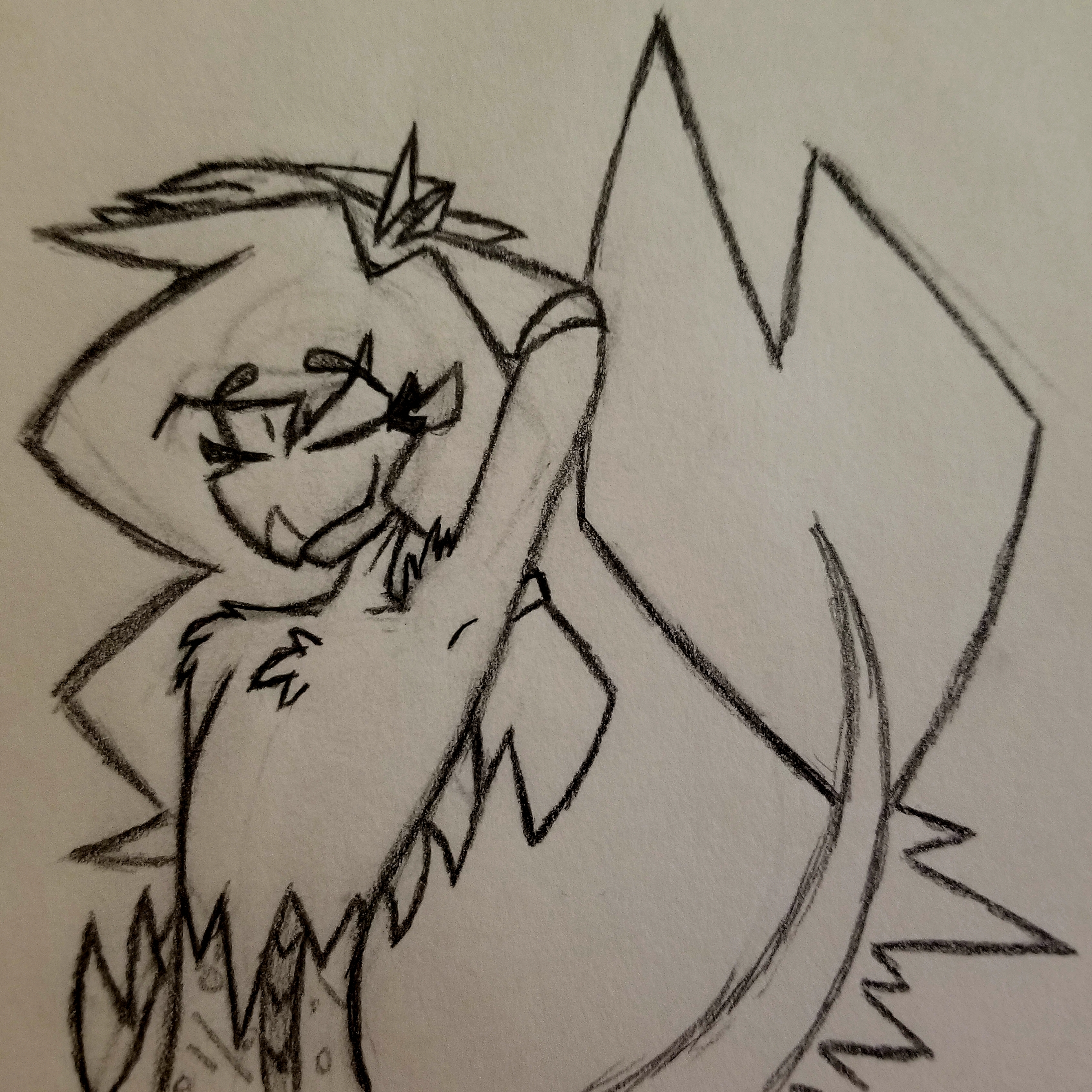
Guillian by King Of All Bees/Chief Bee Officer.
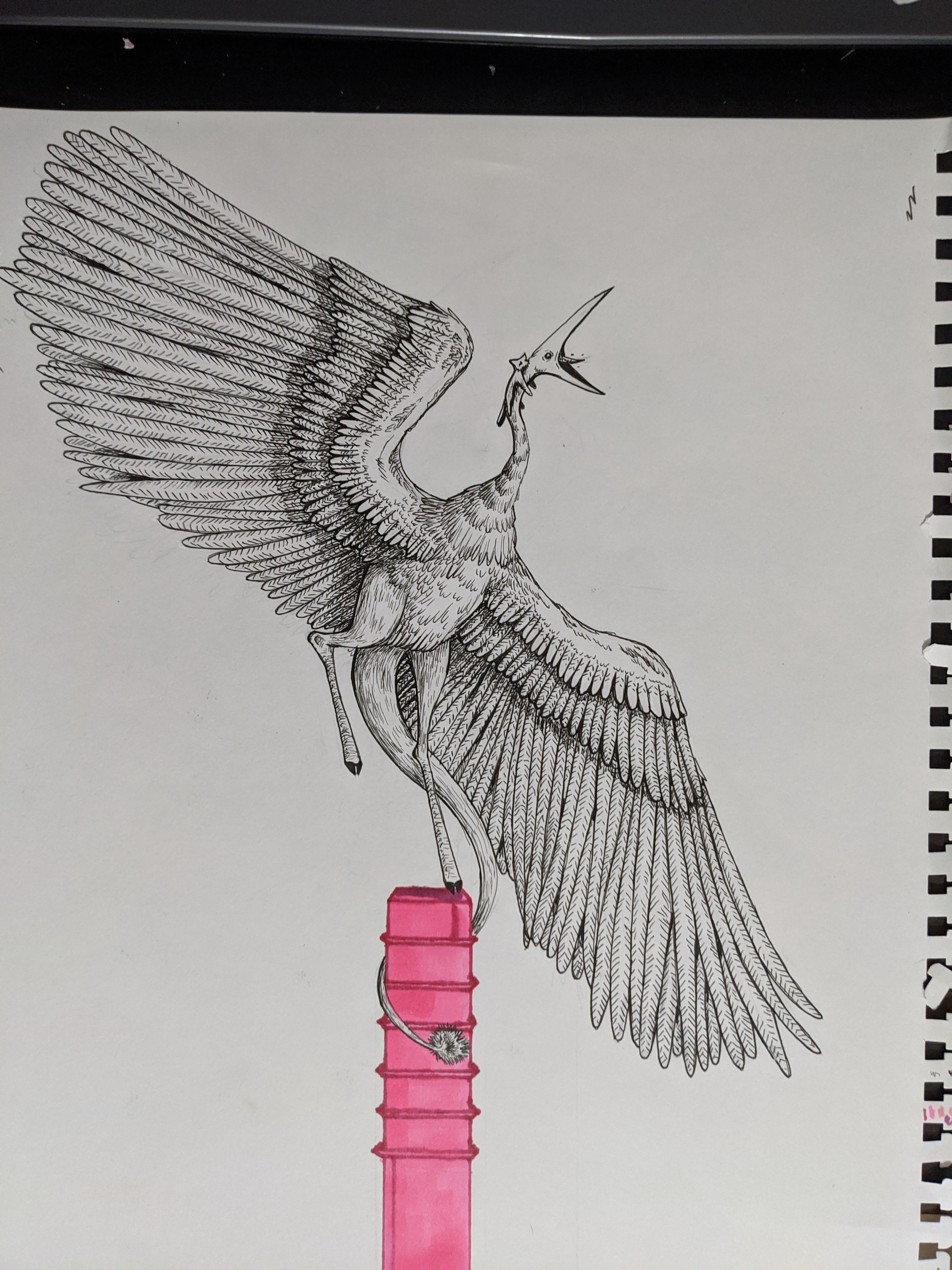
Japhet (From OFF) by Zimzagoon.
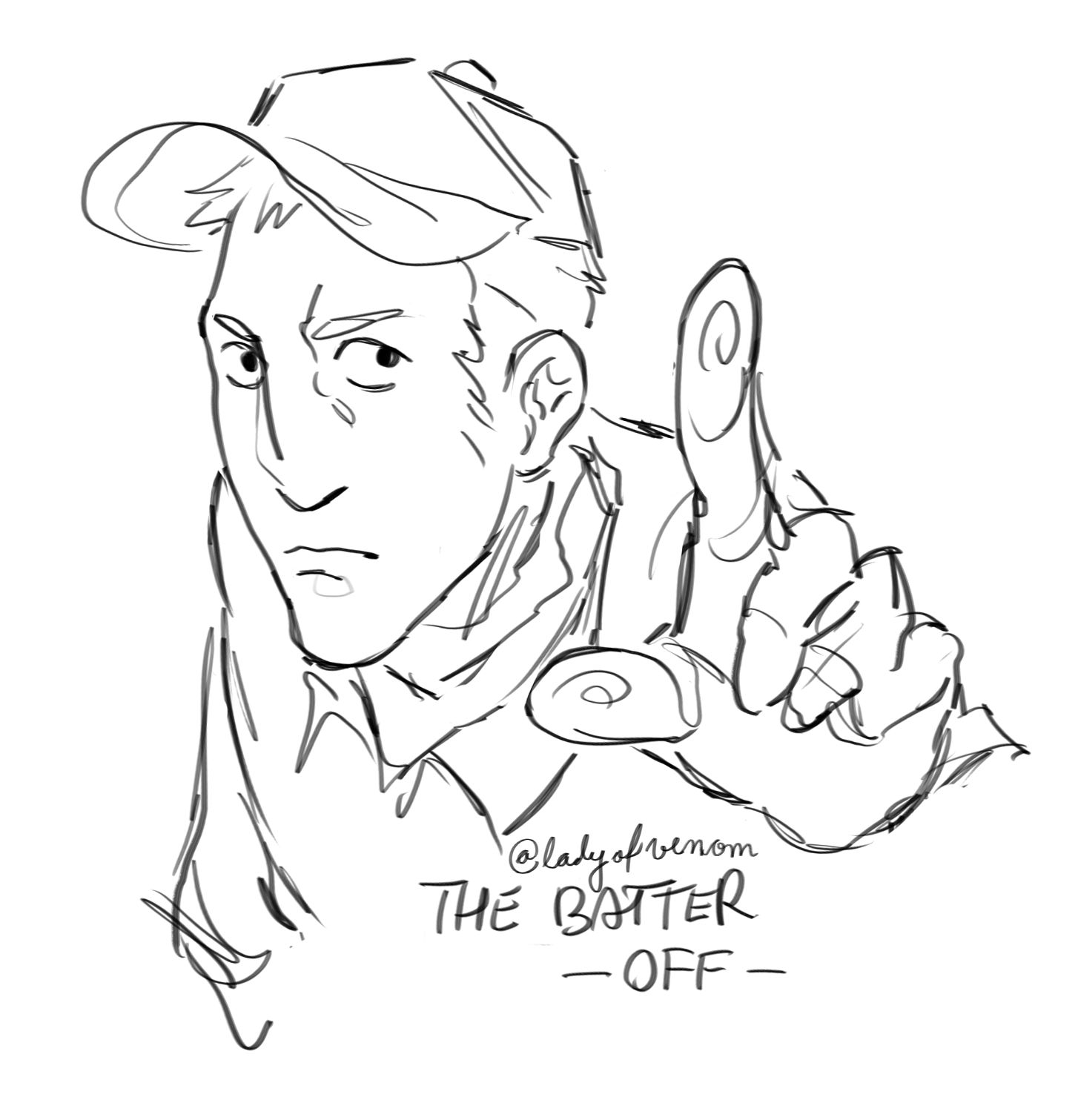
The Batter (From OFF) by VenomAndMercury (@ladyofvenom).
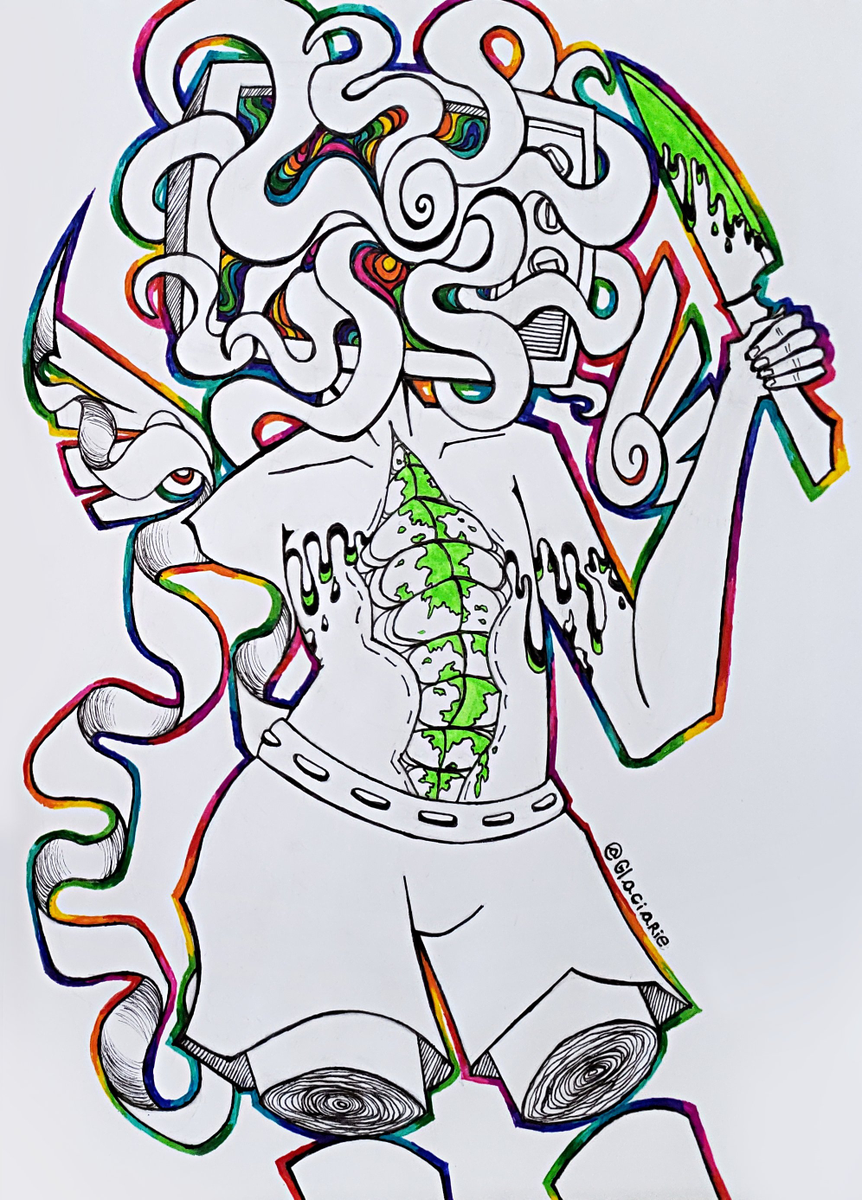
TV Girl by Glaciarie.
The End
Final thoughts.
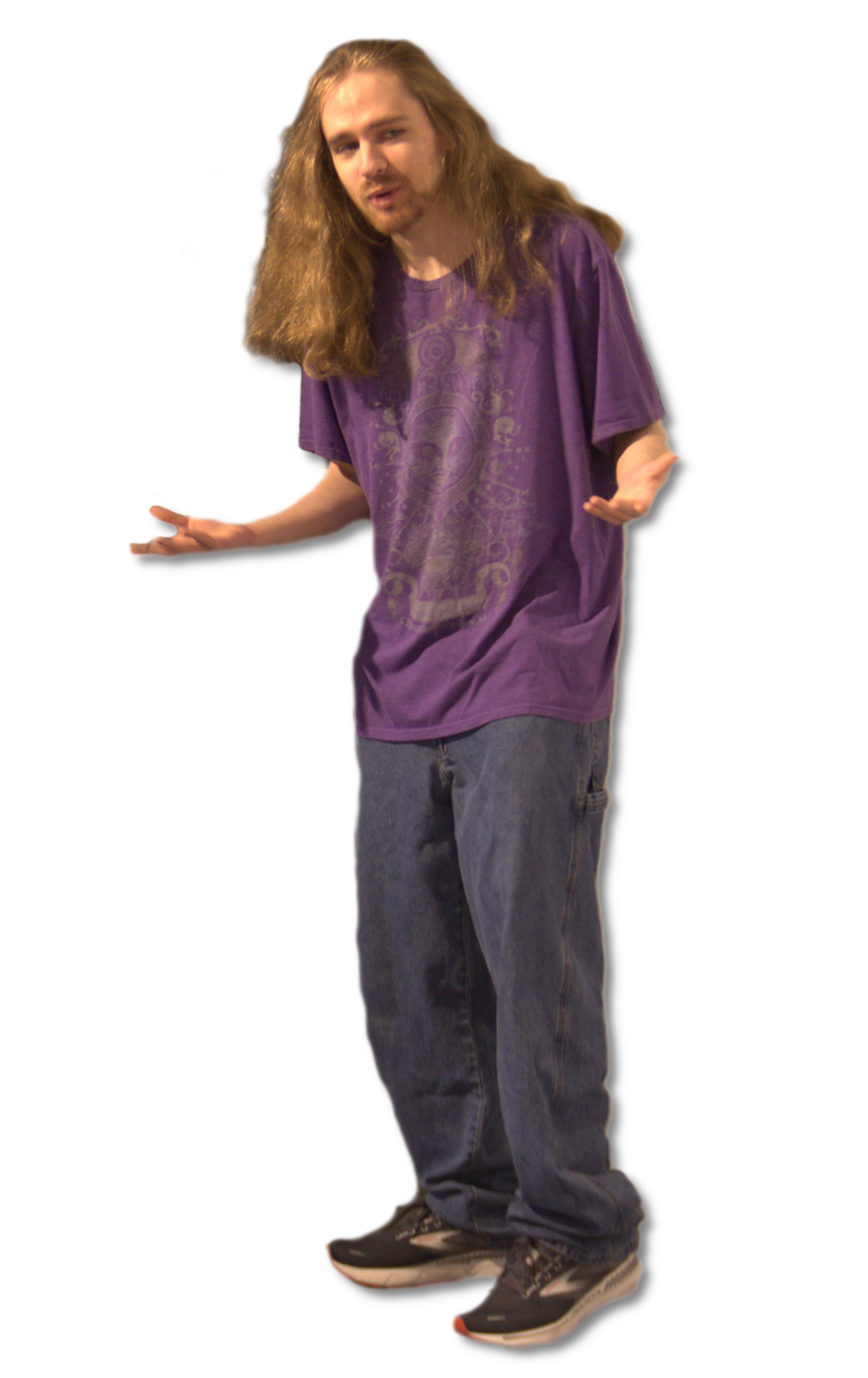
Finite state machines allow us to have more control over our game mechanics in a simple and manageable way by using object oriented design. I hope I did a good job in explaining finite state machines to you, if not then I suggest you read this post from GDQuest.com where I learned how to implement this system. If you have any questions, comments, concerns or testimonies let us know in our Discord.